Frontend technology is evolving rapidly, presenting a challenge of choosing the right one due to their pros and cons. We tend to opt for technologies with minimal risk and maximum benefits. However, what if we could utilize various technologies for different services?
Micro frontends are an architectural approach to building web applications where a single frontend is composed of multiple smaller, independently deployable applications. This concept draws inspiration from microservices. It involves breaking down large frontend monoliths into smaller, more manageable pieces. Each micro frontend is then responsible for a specific feature or functionality within the larger application.
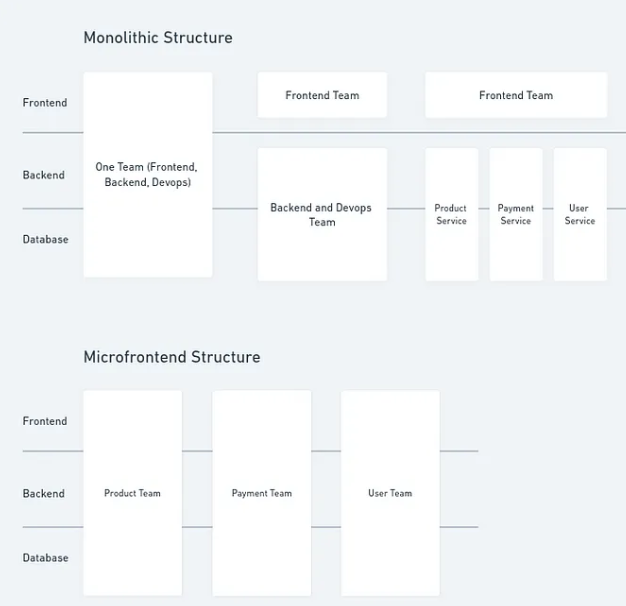
Each team takes charge of a particular specialized part. Micro frontends offer a fresh approach to constructing and scaling frontend apps.
This approach brings several advantages:
- Independent Development and Deployment: With micro frontends, teams can work on different parts of the application independently. This allows for faster development cycles and easier deployment of changes.
- Technology Agnostic: Micro frontends allow teams to use different technologies and frameworks for different parts of the application. This flexibility enables teams to choose the best tool for the job.
- Scalability: Micro frontends make it easier to scale development teams as the application grows. New teams can be added to work on new features without impacting existing teams.
- Isolation and Resilience: Each micro frontend is isolated from the others, which helps in containing failures and preventing them from affecting the entire application.
PS: To sum it all up, we said above that micro-frontends in Angular involve breaking down a large Angular application into smaller, independently deployable applications, each responsible for a specific functionality. This architectural approach allows teams to work independently on different parts of the application, use different technologies for different parts, and scale development more efficiently.
Schema Illustration:
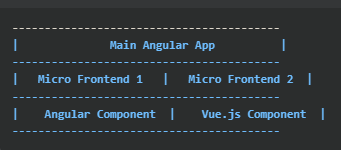
In the schema above, the main Angular application is composed of two micro frontends. Each micro frontend contains a specific component, with one being an Angular component and the other being a Vue.js component.
Code Example:
Here’s a simplified code example demonstrating the concept of micro frontends and mixing Angular with Vue.js in a single application:
- Angular Micro Frontend Component:
// angular-component.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-angular-component',
template: '<h1>This is an Angular Micro Frontend</h1>',
})
export class AngularComponent {}
- Vue.js Micro Frontend Component:
// vue-component.vue
<template>
<div>
<h1>This is a Vue.js Micro Frontend</h1>
</div>
</template>
<script>
export default {
name: 'VueComponent'
}
</script>
- Integration in Main Angular App:
// main.ts (Angular)
import { enableProdMode } from '@angular/core';
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
import { createCustomElement } from '@angular/elements';
import { AngularComponent } from './angular-component.component';
import VueComponent from './vue-component.vue';
enableProdMode();
platformBrowserDynamic().bootstrapModule(AppModule)
.then(moduleRef => {
const angularElement = createCustomElement(AngularComponent, { injector: moduleRef.injector });
customElements.define('angular-component', angularElement);
const vueElement = createCustomElement(VueComponent, { injector: moduleRef.injector });
customElements.define('vue-component', vueElement);
})
.catch(err => console.error(err));
By following this approach, we can implement micro frontends in Angular and mix technologies like Angular and Vue.js within a single application.