I used to struggle with this case, long time ago, how to setup an Angular project generated with Angular CLI with Bootstrap. So let’s see the step by step in the sections below.
1: Creating an Angular project with Angular CLI
ng new angular-bootstrap-example
2: Installing Bootstrap from NPM
For Bootstrap 3:
npm install bootstrap@3.3.7
For Bootstrap 4:
npm install bootstrap
2.1: Alternative: Local Bootstrap CSS
As an alternative, you can also download the Bootstrap CSS and add it locally to your project. let us donwload Bootstrap from the website and create a folder styles
(same level as styles.css
):
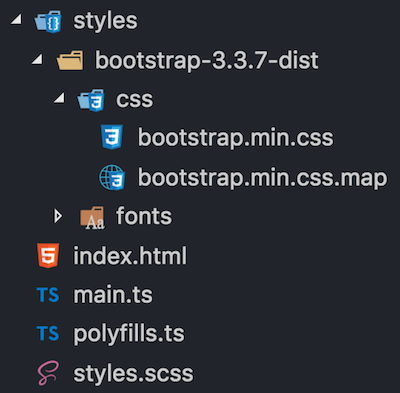
Don’t place your local CSS files under
assets
folder. When we do the production build with Angular CLI, the CSS files declared in theangular.json
will be minified and all styles will be bundled into a single styles.css. The assets folder is copied to the dist folder during the build process (the CSS code will be duplicated). Only place your local CSS files underassets
in case you are importing them directly in theindex.html
.
3: Importing the CSS
We have two options to import the CSS from Bootstrap that was installed from NPM:
1: Configure angular.json
:
"styles": [
"node_modules/bootstrap/dist/css/bootstrap.min.css",
"styles.scss"
]
2: Import directly in src/style.css
or src/style.scss
:
@import '~bootstrap/dist/css/bootstrap.min.css';
3.1 Alternative: Local Bootstrap CSS
If you added the Bootstrap CSS file locally, just import it in angular.json
"styles": [
"styles/bootstrap-3.3.7-dist/css/bootstrap.min.css",
"styles.scss"
],
or import it in src/style.css
:
@import './styles/bootstrap-3.3.7-dist/css/bootstrap.min.css';
Yet, we are able to start using the Bootstrap CSS classes in our project.
In case we don’t need to use Bootstrap JavaScript components (that require JQuery), this is all the setup we need. But if we need to use modals, accordion, datepicker, tooltips or any other component, how can we use these components without installing jQuery?
There is an Angular wrapper library for Bootstrap called ngx-bootstrap that we can also install from NPM:
npm install ngx-bootstrap --save
ng2-bootstrap
andngx-bootstrap
are the same package. ng2-bootstrap was renamed to ngx-bootstrap after#itsJustAngular
.
4: Bootstrap JavaScript Components with ngx-bootstrap
4.1 : Adding the required Bootstrap modules in app.module.ts
Go through the ngx-bootstrap
and add the modules needed in your app.module.ts
. For example, suppose we want to use the Dropdown, Tooltip and Modal components:
mport { BsDropdownModule } from 'ngx-bootstrap/dropdown';
import { TooltipModule } from 'ngx-bootstrap/tooltip';
import { ModalModule } from 'ngx-bootstrap/modal';
@NgModule({
imports: [
BrowserModule,
BsDropdownModule.forRoot(),
TooltipModule.forRoot(),
ModalModule.forRoot()
],
// ...
})
export class AppBootstrapModule {}
Because we call the .forRoot()
method for each module (due the ngx-bootstrap module providers), the functionalities will be available in all components and modules of your project (global scope).
As an alternative, if you would like to organize the ngx-bootstrap
in a different module (just for organization purposes in case you need to import many bs modules and don’t want to clutter your app.module), you can create a module app-bootstrap.module.ts
, import the Bootstrap modules (using forRoot()
) and also declare them in the exports section (so they become available to other modules as well).
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { BsDropdownModule } from 'ngx-bootstrap/dropdown';
import { TooltipModule } from 'ngx-bootstrap/tooltip';
import { ModalModule } from 'ngx-bootstrap/modal';
@NgModule({
imports: [
CommonModule,
BsDropdownModule.forRoot(),
TooltipModule.forRoot(),
ModalModule.forRoot()
],
exports: [BsDropdownModule, TooltipModule, ModalModule]
})
export class AppBootstrapModule {}
At last, don’t forget to import your bootstrap module in you app.module.ts
import { AppBootstrapModule } from './app-bootstrap/app-bootstrap.module';
@NgModule({
imports: [BrowserModule, AppBootstrapModule],
// ...
})
export class AppModule {}
Now, We have an Angular project using Bootstrap and did not need to import JQuery to have the same behavior!